SimPEG.regularization.WeightedLeastSquares#
- class SimPEG.regularization.WeightedLeastSquares(mesh, active_cells=None, alpha_s=1.0, alpha_x=None, alpha_y=None, alpha_z=None, alpha_xx=0.0, alpha_yy=0.0, alpha_zz=0.0, length_scale_x=None, length_scale_y=None, length_scale_z=None, mapping=None, reference_model=None, reference_model_in_smooth=False, weights=None, **kwargs)[source]#
Bases:
ComboObjectiveFunction
Weighted least-squares regularization using smallness and smoothness.
Apply regularization using a weighted sum of
Smallness
,SmoothnessFirstOrder
, and/orSmoothnessSecondOrder
(optional) least-squares regularization functions.Smallness
regularization is used to ensure that values in the recovered model, or differences between the recovered model and a reference model, are not overly large in magnitude.Smoothness
regularization is used to ensure that values in the recovered model are smooth along specified directions. When a reference model is included in the smoothness regularization, the inversion preserves gradients/interfaces within the reference model. Custom weights can also be supplied to control the degree of smallness and smoothness being enforced throughout different regions the model.See the Notes section below for a comprehensive description.
- Parameters:
- mesh
SimPEG.regularization.RegularizationMesh
,discretize.base.BaseMesh
Mesh on which the regularization is discretized. This is not necessarily the same as the mesh on which the simulation is defined.
- active_cells
None
, (n_cells
, )numpy.ndarray
of
bool Boolean array defining the set of
RegularizationMesh
cells that are active in the inversion. IfNone
, all cells are active.- mapping
None
,SimPEG.maps.BaseMap
The mapping from the model parameters to the active cells in the inversion. If
None
, the mapping is the identity map.- reference_model
None
, (n_param
, )numpy.ndarray
Reference model. If
None
, the reference model in the inversion is set to the starting model.- reference_model_in_smoothbool,
optional
Whether to include the reference model in the smoothness terms.
- units
None
,str
Units for the model parameters. Some regularization classes behave differently depending on the units; e.g. ‘radian’.
- weights
None
,dict
Weight multipliers to customize the least-squares function. Each key points to a (n_cells, ) numpy.ndarray that is defined on the
RegularizationMesh
.- alpha_s
float
,optional
Scaling constant for the smallness regularization term.
- alpha_x, alpha_y, alpha_z
float
orNone
,optional
Scaling constants for the first order smoothness along x, y and z, respectively. If set to
None
, the scaling constant is set automatically according to the value of the length_scale parameter.- alpha_xx, alpha_yy, alpha_zz0,
float
Scaling constants for the second order smoothness along x, y and z, respectively. If set to
None
, the scaling constant is set automatically according to the value of the length_scale parameter.- length_scale_x, length_scale_y, length_scale_z
float
,optional
First order smoothness length scales for the respective dimensions.
- mesh
Notes
Weighted least-squares regularization can be defined by a weighted sum of
Smallness
,SmoothnessFirstOrder
andSmoothnessSecondOrder
regularization functions. This corresponds to a model objective function \(\phi_m (m)\) of the form:\[\begin{split}\phi_m (m) =& \alpha_s \int_\Omega \, w(r) \Big [ m(r) - m^{(ref)}(r) \Big ]^2 \, dv \\ &+ \sum_{j=x,y,z} \alpha_j \int_\Omega \, w(r) \bigg [ \frac{\partial m}{\partial \xi_j} \bigg ]^2 \, dv \\ &+ \sum_{j=x,y,z} \alpha_{jj} \int_\Omega \, w(r) \bigg [ \frac{\partial^2 m}{\partial \xi_j^2} \bigg ]^2 \, dv \;\;\;\;\;\;\;\; \big ( \textrm{optional} \big )\end{split}\]where \(m(r)\) is the model, \(m^{(ref)}(r)\) is the reference model, and \(w(r)\) is a user-defined weighting function. \(\xi_j\) is the unit direction along \(j\). Parameters \(\alpha_s\), \(\alpha_j\) and \(\alpha_{jj}\) for \(j=x,y,z\) are multiplier constants which weight the respective contributions of the smallness and smoothness terms towards the regularization.
For implementation within SimPEG, the regularization functions and their variables must be discretized onto a mesh. For a continuous variable \(x(r)\) whose discrete representation on the mesh is given by \(\mathbf{x}\), we approximate as follows:
\[\int_\Omega w(r) \big [ x(r) \big ]^2 \, dv \approx \sum_i \tilde{w}_i \, | x_i |^2\]where \(\tilde{w}_i\) are amalgamated weighting constants that account for cell dimensions in the discretization and apply user-defined weighting. Using the above approximation, the
WeightedLeastSquares
regularization can be expressed as a weighted sum of objective functions of the form:\[\begin{split}\phi_m (\mathbf{m}) =& \alpha_s \Big \| \mathbf{W_s} \big [ \mathbf{m} - \mathbf{m}^{(ref)} \big ] \Big \|^2 \\ &+ \sum_{j=x,y,z} \alpha_j \Big \| \mathbf{W_j G_j \, m} \, \Big \|^2 \\ &+ \sum_{j=x,y,z} \alpha_{jj} \Big \| \mathbf{W_{jj} L_j \, m} \, \Big \|^2 \;\;\;\;\;\;\;\; \big ( \textrm{optional} \big )\end{split}\]where
\(\mathbf{m}\) are the set of discrete model parameters (i.e. the model),
\(\mathbf{m}^{(ref)}\) is the reference model,
\(\mathbf{G_x, \, G_y, \; G_z}\) are partial cell gradients operators along x, y and z,
\(\mathbf{L_x, \, L_y, \; L_z}\) are second-order derivative operators with respect to x, y and z,
\(\mathbf{W_s, \, W_x, \, W_y, \; W_z}\) are weighting matrices.
Reference model in smoothness:
Gradients/interfaces within a discrete reference model \(\mathbf{m}^{(ref)}\) can be preserved by including the reference model the smoothness regularization. In this case, the objective function becomes:
\[\begin{split}\phi_m (\mathbf{m}) =& \alpha_s \Big \| \mathbf{W_s} \big [ \mathbf{m} - \mathbf{m}^{(ref)} \big ] \Big \|^2 \\ &+ \sum_{j=x,y,z} \alpha_j \Big \| \mathbf{W_j G_j} \big [ \mathbf{m} - \mathbf{m}^{(ref)} \big ] \Big \|^2 \\ &+ \sum_{j=x,y,z} \alpha_{jj} \Big \| \mathbf{W_{jj} L_j} \big [ \mathbf{m} - \mathbf{m}^{(ref)} \big ] \Big \|^2 \;\;\;\;\;\;\;\; \big ( \textrm{optional} \big )\end{split}\]This functionality is used by setting the reference_model_in_smooth parameter to
True
.Alphas and length scales:
The \(\alpha\) parameters scale the relative contributions of the smallness and smoothness terms in the model objective function. Each \(\alpha\) parameter can be set directly as a appropriate property of the
WeightedLeastSquares
class; e.g. \(\alpha_x\) is set using the alpha_x property. Note that unless the parameters are set manually, second-order smoothness is not included in the model objective function. That is, the alpha_xx, alpha_yy and alpha_zz parameters are set to 0 by default.The relative contributions of smallness and smoothness terms on the recovered model can also be set by leaving alpha_s as its default value of 1, and setting the smoothness scaling constants based on length scales. The model objective function has been formulated such that smallness and smoothness terms contribute equally when the length scales are equal; i.e. when properties length_scale_x = length_scale_y = length_scale_z. When the length_scale_x property is set, the alpha_x and alpha_xx properties are set internally as:
>>> reg.alpha_x = (reg.length_scale_x * reg.regularization_mesh.base_length) ** 2.0
and
>>> reg.alpha_xx = (ref.length_scale_x * reg.regularization_mesh.base_length) ** 4.0
Likewise for y and z.
Custom weights and weighting matrices:
Let \(\mathbf{w_1, \; w_2, \; w_3, \; ...}\) each represent an optional set of custom cell weights that are applied to all terms in the model objective function. The general form for the weights applied to smallness and second-order smoothness terms is given by:
\[\mathbf{\tilde{w}} = \mathbf{v} \odot \prod_j \mathbf{w_j}\]and weights applied to first-order smoothness terms are given by:
\[\mathbf{\tilde{w}} = \big ( \mathbf{P \, v} \big ) \odot \prod_j \mathbf{P \, w_j}\]\(\mathbf{v}\) are the cell volumes, and \(\mathbf{P}\) represents the projection matrix from cell centers to the appropriate faces; i.e. where discrete first-order derivatives live.
Weights for each term are used to construct their respective weighting matrices as follows:
\[\mathbf{W} = \textrm{diag} \Big ( \, \mathbf{\tilde{w}}^{1/2} \Big )\]Each set of custom cell weights is stored within a
dict
as an (n_cells, )numpy.ndarray
. The weights can be set all at once during instantiation with the weights keyword argument as follows:>>> reg = WeightedLeastSquares(mesh, weights={'weights_1': array_1, 'weights_2': array_2})
or set after instantiation using the set_weights method:
>>> reg.set_weights(weights_1=array_1, weights_2=array_2})
Attributes
Full weighting matrix for the combo objective function.
Active cells defined on the regularization mesh.
Multiplier constant for the smallness term.
Multiplier constant for first-order smoothness along x.
Multiplier constant for second-order smoothness along x.
Multiplier constant for first-order smoothness along y.
Multiplier constant for second-order smoothness along y.
Multiplier constant for first-order smoothness along z.
Multiplier constant for second-order smoothness along z.
active_cells.indActive has been deprecated.
Multiplier constant for smoothness along x relative to base scale length.
Multiplier constant for smoothness along z relative to base scale length.
Multiplier constant for smoothness along z relative to base scale length.
Mapping from the model to the regularization mesh.
The model associated with regularization.
reference_model.mref has been deprecated.
Multiplier constants for weighted sum of objective functions.
Number of model parameters.
Reference model.
Whether to include the reference model in the smoothness objective functions.
Regularization mesh.
Units for the model parameters.
cell_weights
Methods
__call__
(m[, f])Evaluate the objective functions for a given model.
deriv
(m[, f])Gradient of the objective function evaluated for the model provided.
deriv2
(m[, v, f])Hessian of the objective function evaluated for the model provided.
get_functions_of_type
(fun_class)Return objective functions of a given type(s).
map_class
alias of
IdentityMap
remove_weights
(key)Removes specified weights from all child regularization objects.
set_weights
(**weights)Adds (or updates) the specified weights for all child regularization objects.
test
([x, num])Run a convergence test on both the first and second derivatives.
Galleries and Tutorials using SimPEG.regularization.WeightedLeastSquares
#
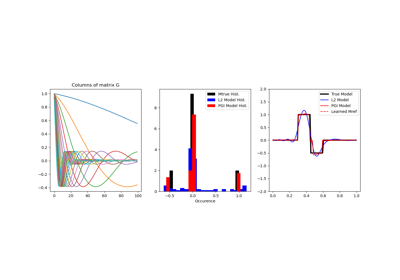
Petrophysically guided inversion (PGI): Linear example
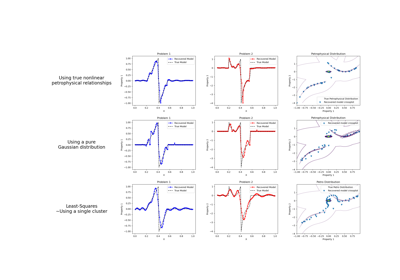
Petrophysically guided inversion: Joint linear example with nonlinear relationships
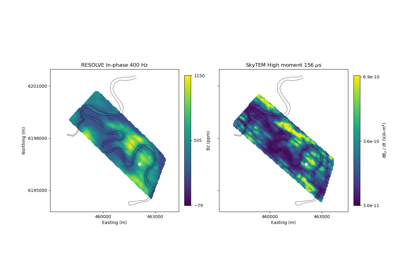
Heagy et al., 2017 1D RESOLVE and SkyTEM Bookpurnong Inversions
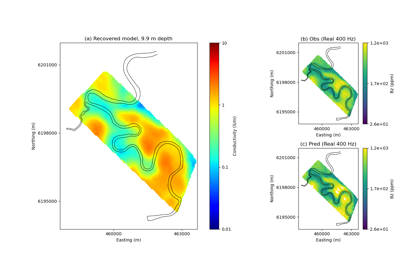
Heagy et al., 2017 1D RESOLVE Bookpurnong Inversion
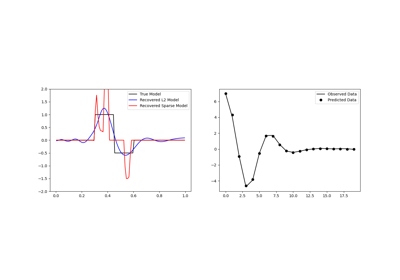
Sparse Inversion with Iteratively Re-Weighted Least-Squares
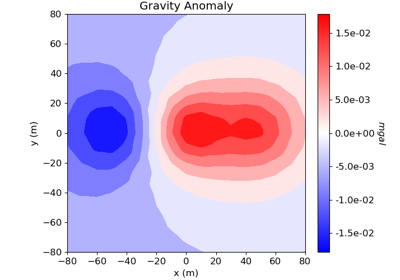
Cross-gradient Joint Inversion of Gravity and Magnetic Anomaly Data
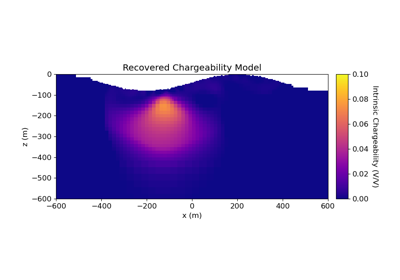
2.5D DC Resistivity and IP Least-Squares Inversion
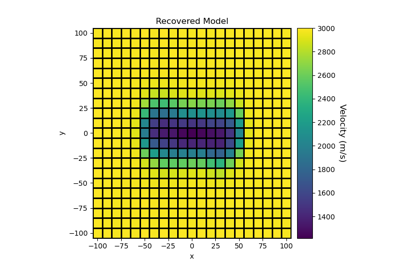
Sparse Norm Inversion of 2D Seismic Tomography Data
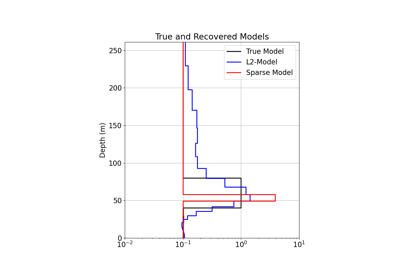
1D Inversion of Time-Domain Data for a Single Sounding
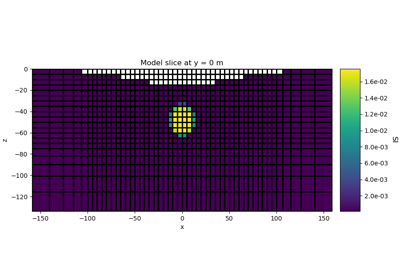
Sparse Norm Inversion for Total Magnetic Intensity Data on a Tensor Mesh