Note
Go to the end to download the full example code.
Petrophysically guided inversion (PGI): Linear example#
We do a comparison between the classic least-squares inversion and our formulation of a petrophysically constrained inversion. We explore it through the UBC linear example.
Tikhonov Inversion##
import discretize as Mesh
import matplotlib.pyplot as plt
import numpy as np
from simpeg import (
data_misfit,
directives,
inverse_problem,
inversion,
maps,
optimization,
regularization,
simulation,
utils,
)
# Random seed for reproductibility
np.random.seed(1)
# Mesh
N = 100
mesh = Mesh.TensorMesh([N])
# Survey design parameters
nk = 20
jk = np.linspace(1.0, 60.0, nk)
p = -0.25
q = 0.25
# Physics
def g(k):
return np.exp(p * jk[k] * mesh.cell_centers_x) * np.cos(
np.pi * q * jk[k] * mesh.cell_centers_x
)
G = np.empty((nk, mesh.nC))
for i in range(nk):
G[i, :] = g(i)
# True model
mtrue = np.zeros(mesh.nC)
mtrue[mesh.cell_centers_x > 0.2] = 1.0
mtrue[mesh.cell_centers_x > 0.35] = 0.0
t = (mesh.cell_centers_x - 0.65) / 0.25
indx = np.abs(t) < 1
mtrue[indx] = -(((1 - t**2.0) ** 2.0)[indx])
mtrue = np.zeros(mesh.nC)
mtrue[mesh.cell_centers_x > 0.3] = 1.0
mtrue[mesh.cell_centers_x > 0.45] = -0.5
mtrue[mesh.cell_centers_x > 0.6] = 0
# simpeg problem and survey
prob = simulation.LinearSimulation(mesh, G=G, model_map=maps.IdentityMap())
std = 0.01
survey = prob.make_synthetic_data(mtrue, relative_error=std, add_noise=True)
# Setup the inverse problem
reg = regularization.WeightedLeastSquares(mesh, alpha_s=1.0, alpha_x=1.0)
dmis = data_misfit.L2DataMisfit(data=survey, simulation=prob)
opt = optimization.ProjectedGNCG(maxIter=10, maxIterCG=50, tolCG=1e-4)
invProb = inverse_problem.BaseInvProblem(dmis, reg, opt)
directiveslist = [
directives.BetaEstimate_ByEig(beta0_ratio=1e-5),
directives.BetaSchedule(coolingFactor=10.0, coolingRate=2),
directives.TargetMisfit(),
]
inv = inversion.BaseInversion(invProb, directiveList=directiveslist)
m0 = np.zeros_like(mtrue)
mnormal = inv.run(m0)
Running inversion with SimPEG v0.22.2.dev13+g048ef809f
simpeg.InvProblem will set Regularization.reference_model to m0.
simpeg.InvProblem will set Regularization.reference_model to m0.
simpeg.InvProblem will set Regularization.reference_model to m0.
simpeg.InvProblem is setting bfgsH0 to the inverse of the eval2Deriv.
***Done using the default solver Pardiso and no solver_opts.***
model has any nan: 0
=============================== Projected GNCG ===============================
# beta phi_d phi_m f |proj(x-g)-x| LS Comment
-----------------------------------------------------------------------------
x0 has any nan: 0
0 1.83e+01 2.00e+05 0.00e+00 2.00e+05 2.45e+06 0
1 1.83e+01 3.97e+01 4.63e+01 8.87e+02 2.20e-05 0
------------------------- STOP! -------------------------
0 : |fc-fOld| = 1.9911e+05 <= tolF*(1+|f0|) = 2.0000e+04
0 : |xc-x_last| = 3.9921e+00 <= tolX*(1+|x0|) = 1.0000e-01
1 : |proj(x-g)-x| = 2.2041e-05 <= tolG = 1.0000e-01
1 : |proj(x-g)-x| = 2.2041e-05 <= 1e3*eps = 1.0000e-02
0 : maxIter = 10 <= iter = 1
------------------------- DONE! -------------------------
Petrophysically constrained inversion ##
# fit a Gaussian Mixture Model with n components
# on the true model to simulate the laboratory
# petrophysical measurements
n = 3
clf = utils.WeightedGaussianMixture(
mesh=mesh,
n_components=n,
covariance_type="full",
max_iter=100,
n_init=3,
reg_covar=5e-4,
)
clf.fit(mtrue.reshape(-1, 1))
# Petrophyically constrained regularization
reg = regularization.PGI(
gmmref=clf,
mesh=mesh,
alpha_pgi=1.0,
alpha_x=1.0,
)
# Optimization
opt = optimization.ProjectedGNCG(maxIter=20, maxIterCG=50, tolCG=1e-4)
opt.remember("xc")
# Setup new inverse problem
invProb = inverse_problem.BaseInvProblem(dmis, reg, opt)
# directives
Alphas = directives.AlphasSmoothEstimate_ByEig(alpha0_ratio=10.0, verbose=True)
beta = directives.BetaEstimate_ByEig(beta0_ratio=1e-8)
betaIt = directives.PGI_BetaAlphaSchedule(
verbose=True,
coolingFactor=2.0,
warmingFactor=1.0,
tolerance=0.1,
update_rate=1,
progress=0.2,
)
targets = directives.MultiTargetMisfits(verbose=True)
petrodir = directives.PGI_UpdateParameters()
addmref = directives.PGI_AddMrefInSmooth(verbose=True)
# Setup Inversion
inv = inversion.BaseInversion(
invProb, directiveList=[Alphas, beta, petrodir, targets, addmref, betaIt]
)
# Initial model same as for WeightedLeastSquares
mcluster = inv.run(m0)
# Final Plot
fig, axes = plt.subplots(1, 3, figsize=(12 * 1.2, 4 * 1.2))
for i in range(prob.G.shape[0]):
axes[0].plot(prob.G[i, :])
axes[0].set_title("Columns of matrix G")
axes[1].hist(mtrue, bins=20, linewidth=3.0, density=True, color="k")
axes[1].set_xlabel("Model value")
axes[1].set_xlabel("Occurence")
axes[1].hist(mnormal, bins=20, density=True, color="b")
axes[1].hist(mcluster, bins=20, density=True, color="r")
axes[1].legend(["Mtrue Hist.", "L2 Model Hist.", "PGI Model Hist."])
axes[2].plot(mesh.cell_centers_x, mtrue, color="black", linewidth=3)
axes[2].plot(mesh.cell_centers_x, mnormal, color="blue")
axes[2].plot(mesh.cell_centers_x, mcluster, "r-")
axes[2].plot(mesh.cell_centers_x, invProb.reg.objfcts[0].reference_model, "r--")
axes[2].legend(("True Model", "L2 Model", "PGI Model", "Learned Mref"))
axes[2].set_ylim([-2, 2])
plt.show()
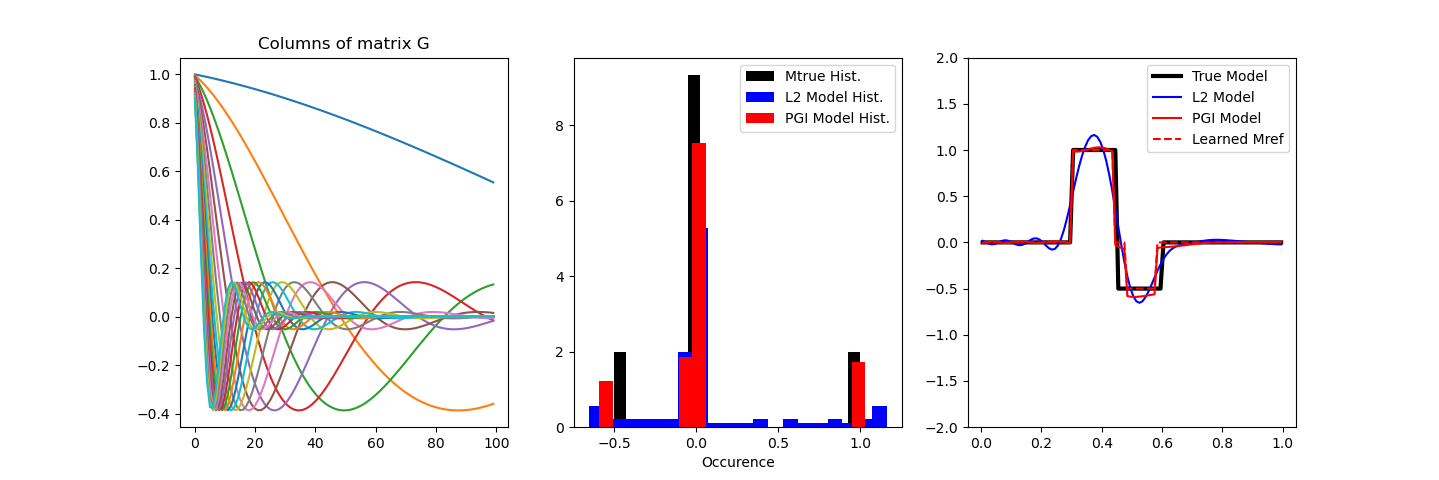
Running inversion with SimPEG v0.22.2.dev13+g048ef809f
simpeg.InvProblem will set Regularization.reference_model to m0.
simpeg.InvProblem will set Regularization.reference_model to m0.
simpeg.InvProblem is setting bfgsH0 to the inverse of the eval2Deriv.
***Done using the default solver Pardiso and no solver_opts.***
Alpha scales: [0.5314568789240659, 0.0]
<class 'simpeg.regularization.pgi.PGIsmallness'>
model has any nan: 0
=============================== Projected GNCG ===============================
# beta phi_d phi_m f |proj(x-g)-x| LS Comment
-----------------------------------------------------------------------------
x0 has any nan: 0
0 3.24e-02 2.00e+05 0.00e+00 2.00e+05 2.45e+06 0
geophys. misfits: 5.1 (target 20.0 [True]) | smallness misfit: 3677.5 (target: 100.0 [False])
mref changed in 26 places
Beta cooling evaluation: progress: [5.1]; minimum progress targets: [160000.]
Warming alpha_pgi to favor clustering: 3.9019428837247996
1 3.24e-02 5.13e+00 1.73e+02 1.07e+01 8.05e+00 0
geophys. misfits: 5.4 (target 20.0 [True]) | smallness misfit: 1088.7 (target: 100.0 [False])
mref changed in 0 places
Add mref to Smoothness. Changes in mref happened in 0.0 % of the cells
Beta cooling evaluation: progress: [5.4]; minimum progress targets: [22.]
Warming alpha_pgi to favor clustering: 14.529046331064658
2 3.24e-02 5.37e+00 2.08e+02 1.21e+01 1.03e+01 0 Skip BFGS
geophys. misfits: 6.1 (target 20.0 [True]) | smallness misfit: 358.5 (target: 100.0 [False])
mref changed in 0 places
Add mref to Smoothness. Changes in mref happened in 0.0 % of the cells
Beta cooling evaluation: progress: [6.1]; minimum progress targets: [22.]
Warming alpha_pgi to favor clustering: 47.844882418020774
3 3.24e-02 6.07e+00 2.54e+02 1.43e+01 5.76e+02 0
geophys. misfits: 7.0 (target 20.0 [True]) | smallness misfit: 118.1 (target: 100.0 [False])
mref changed in 0 places
Add mref to Smoothness. Changes in mref happened in 0.0 % of the cells
Beta cooling evaluation: progress: [7.]; minimum progress targets: [22.]
Warming alpha_pgi to favor clustering: 136.45817949488588
4 3.24e-02 7.01e+00 2.72e+02 1.58e+01 3.28e+01 0
geophys. misfits: 7.7 (target 20.0 [True]) | smallness misfit: 70.4 (target: 100.0 [True])
All targets have been reached
mref changed in 0 places
Add mref to Smoothness. Changes in mref happened in 0.0 % of the cells
Beta cooling evaluation: progress: [7.7]; minimum progress targets: [22.]
Warming alpha_pgi to favor clustering: 354.17258386707084
------------------------- STOP! -------------------------
1 : |fc-fOld| = 0.0000e+00 <= tolF*(1+|f0|) = 2.0000e+04
1 : |xc-x_last| = 9.7660e-02 <= tolX*(1+|x0|) = 1.0000e-01
0 : |proj(x-g)-x| = 3.2758e+01 <= tolG = 1.0000e-01
0 : |proj(x-g)-x| = 3.2758e+01 <= 1e3*eps = 1.0000e-02
0 : maxIter = 20 <= iter = 5
------------------------- DONE! -------------------------
Total running time of the script: (0 minutes 5.467 seconds)
Estimated memory usage: 235 MB