SimPEG.regularization.CrossGradient#
- class SimPEG.regularization.CrossGradient(mesh, wire_map, approx_hessian=True, **kwargs)[source]#
Bases:
BaseSimilarityMeasure
Cross-gradient regularization for joint inversion.
CrossGradient
regularization is used to ensure the location and orientation of non-zero gradients in the recovered model are consistent across two physical property distributions. For joint inversion involving three or more physical properties, a separate instance ofCrossGradient
must be created for each physical property pair and added to the total regularization as a weighted sum.- Parameters:
- mesh
SimPEG.regularization.RegularizationMesh
,discretize.base.BaseMesh
Mesh on which the regularization is discretized. This is not necessarily the same as the mesh on which the simulation is defined.
- active_cells
None
, (n_cells
, )numpy.ndarray
of
bool Boolean array defining the set of
RegularizationMesh
cells that are active in the inversion. IfNone
, all cells are active.- wire_map
SimPEG.maps.Wires
Wire map connecting physical properties defined on active cells of the
RegularizationMesh`
to the entire model.- reference_model
None
, (n_param
, )numpy.ndarray
Reference model. If
None
, the reference model in the inversion is set to the starting model.- units
None
,str
Units for the model parameters. Some regularization classes behave differently depending on the units; e.g. ‘radian’.
- weights
None
,dict
Weight multipliers to customize the least-squares function. Each key points to a (n_cells, ) numpy.ndarray that is defined on the
RegularizationMesh
.- approx_hessianbool
Whether to use the semi-positive definate approximation for the Hessian.
- mesh
Notes
Consider the case where the model is comprised of two physical properties
and . Here, we define the regularization function (objective function) for cross-gradient as (Haber and Gazit, 2013):where
is a user-defined weighting function. Using the identity , the regularization function can be re-expressed as:For implementation within SimPEG, the regularization function and its variables must be discretized onto a mesh. The discretized approximation for the regularization function (objective function) is given by:
where
are the gradients of property defined on the mesh and are amalgamated weighting constants that 1) account for cell dimensions in the discretization and 2) apply any user-defined weighting.In practice, we define the model
as a discrete vector of the form:where
and are the discrete representations of the respective physical properties on the mesh. The discrete regularization function is therefore equivalent to an objective function of the form:where exponents are computed elementwise,
is the cell gradient operator (cell centers to faces), averages vectors from faces to cell centers, and is the weighting matrix.
Custom weights and the weighting matrix:
Let
each represent an optional set of custom cell weights. The weighting applied within the objective function is given by:where
are the cell volumes. The weighting matrix used to apply weights within the regularization is given by:Each set of custom cell weights is stored within a
dict
as an (n_cells, )numpy.ndarray
. The weights can be set all at once during instantiation with the weights keyword argument as follows:>>> reg = CrossGradient(mesh, wire_map, weights={'weights_1': array_1, 'weights_2': array_2})
or set after instantiation using the set_weights method:
>>> reg.set_weights(weights_1=array_1, weights_2=array_2})
The default weights that account for cell dimensions in the regularization are accessed via:
>>> reg.get_weights('volume')
Attributes
Whether to use the semi-positive definate approximation for the Hessian.
Methods
__call__
(model)Evaluate the cross-gradient regularization function for the model provided.
calculate_cross_gradient
(model[, ...])Calculates the magnitudes of the cross-gradient vectors at cell centers.
deriv
(model)Gradient of the regularization function evaluated for the model provided.
deriv2
(model[, v])Hessian of the regularization function evaluated for the model provided.
Galleries and Tutorials using SimPEG.regularization.CrossGradient
#
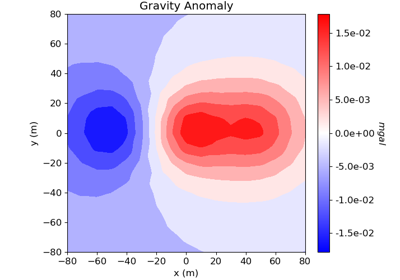
Cross-gradient Joint Inversion of Gravity and Magnetic Anomaly Data