simpeg.maps.Wires#
- class simpeg.maps.Wires(*args)[source]#
Bases:
object
Mapping class for organizing multiple parameter types into a single model.
Let
and be vectors that contain the parameter values for two different parameter types; for example, electrical conductivity and magnetic permeability. Here, all parameters are organized into a single model of the form:The
Wires
class constructs and applies the basic projection mappings for extracting the values of a particular parameter type from the model. For example:where
is the projection matrix that extracts parameters from the complete set of model parameters . Likewise, there is a projection matrix for extracting . This can be extended to a model that containing more than 2 parameter types.- Parameters:
- args
tuple
Each input argument is a tuple (
str
,int
) that provides the name and number of parameters for a given parameters type.
- args
Attributes
Number of parameters the mapping acts on.
Examples
Here we construct a wire mapping for a model where there are two parameters types. Note that the number of parameters of each type does not need to be the same.
>>> from simpeg.maps import Wires, ReciprocalMap >>> import numpy as np
>>> p1 = np.r_[4.5, 2.7, 6.9, 7.1, 1.2] >>> p2 = np.r_[10., 2., 5.]**-1 >>> nP1 = len(p1) >>> nP2 = len(p2) >>> m = np.r_[p1, p2] >>> m array([4.5, 2.7, 6.9, 7.1, 1.2, 0.1, 0.5, 0.2])
Here we construct the wire map. The user provides a name and the number of parameters for each type. The name provided becomes the name of the method for constructing the projection mapping.
>>> wire_map = Wires(('name_1', nP1), ('name_2', nP2))
Here, we extract the values for the first parameter type.
>>> wire_map.name_1 * m array([4.5, 2.7, 6.9, 7.1, 1.2])
And here, we extract the values for the second parameter type then apply a reciprocal mapping.
>>> reciprocal_map = ReciprocalMap() >>> reciprocal_map * wire_map.name_2 * m array([10., 2., 5.])
Galleries and Tutorials using simpeg.maps.Wires
#

Petrophysically guided inversion: Joint linear example with nonlinear relationships
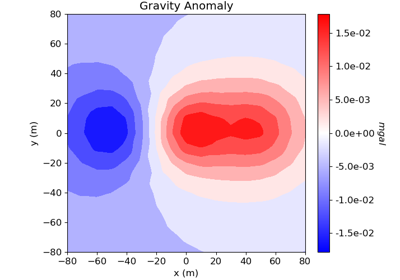
Cross-gradient Joint Inversion of Gravity and Magnetic Anomaly Data

Joint PGI of Gravity + Magnetic on an Octree mesh using full petrophysical information

Joint PGI of Gravity + Magnetic on an Octree mesh without petrophysical information